This cheat sheet is the most concise Python cheat sheet in the world. It contains keywords, basic data structures, and complex data structures—all in a single 1-page PDF file. If you’re lazy, this cheat sheet is a must! If you love cheat sheets, here are some interesting references for you (lots of more PDF downloads). String (converts any Python object using repr. 's' String (converts any Python object using str '%' No argument is converted, results in a '%' character in the. Shortcuts Start Debugging F5 Step Over F10 Step Into F11 Step Out Shift+F11 Stop Debugging Shift+F5 DEBUGGING CODING (Windows) CODING (Mac) End of line fn+Right. Do you want to learn Python but you're overwhelmed and you don't know where to start? Learn with Python cheat sheets! They compress the most important information in an easy-to-digest 1-page format. Here's the new Python cheat sheet I just created-my goal was to make it the world's most concise Python cheat sheet! Download PDF The World’s Most Concise Python Cheat Sheet Read More ».
Resource
Download
String
String Methods
File
Methods
Attributes
Set & Mapping
Set Types
- len(s)
- x in s
- x not in s
Mapping Types
- len(d)
- d[key]
- d[key] = value
- del d[key]
- key in d
- key not in d
- iter(d)
Date Time
Date Object
Datetime Object
Time Object
Array
Array Methods
Indexes and Slices
- a=[0,1,2,3,4,5]
- 6
- len(a)
- 0
- a[0]
- 5
- a[5]
- 5
- a[-1]
- 4
- a[-2]
- [1,2,3,4,5]
- a[1:]
- [0,1,2,3,4]
- a[:5]
- [0,1,2,3]
- a[:-2]
- [1,2]
- a[1:3]
- [1,2,3,4]
- a[1:-1]
- Shallow copy of a
- b=a[:]
Math
Number Theoretic
Power and Logarithmic
Trigonometric Functions
Hyperbolic Functions
Constants
- math.pi
- The mathematical constant π = 3.141592..., to available precision.
- math.e
- The mathematical constant e = 2.718281..., to available precision.
Random
Functions
Sys
Sys Variables
- argv
- Command line args
- builtin_module_names
- Linked C modules
- byteorder
- Native byte order
- check_-interval
- Signal check frequency
- exec_prefix
- Root directory
- executable
- Name of executable
- exitfunc
- Exit function name
- modules
- Loaded modules
- path
- Search path
- platform
- Current platform
- stdin, stdout, stderr
- File objects for I/O
- version_info
- Python version info
- winver
- Version number
sys.argv
- foo.py
- sys.argv[0]
- bar
- sys.argv[1]
- -c
- sys.argv[2]
- qux
- sys.argv[3]
- --h
- sys.argv[4]
OS

os Variables
- altsep
- Alternative sep
- curdir
- Current dir string
- defpath
- Default search path
- devnull
- Path of null device
- extsep
- Extension separator
- linesep
- Line separator
- name
- Name of OS
- pardir
- Parent dir string
- pathsep
- Patch separator
- sep
- Path separator
Class
Special Methods
- __new__(cls)
- __lt__(self, other)
- __init__(self, args)
- __le__(self, other)
- __del__(self)
- __gt__(self, other)
- __repr__(self)
- __ge__(self, other)
- __str__(self)
- __eq__(self, other)
- __cmp__(self, other)
- __ne__(self, other)
- __index__(self)
- __nonzero__(self)
- __hash__(self)
- __getattr__(self, name)
- __getattribute__(self, name)
- __setattr__(self, name, attr)
- __delattr__(self, name)
- __call__(self, args, kwargs)
String Formatting
Formatting Operations
- 'd'
- Signed integer decimal.
- 'i'
- Signed integer decimal.
- 'o'
- Signed octal value.
- 'u'
- Obsolete type – it is identical to 'd'.
- 'x'
- Signed hexadecimal (lowercase).
- 'X'
- Signed hexadecimal (uppercase).
- 'e'
- Floating point exponential format (lowercase).
- 'E'
- Floating point exponential format (uppercase).
- 'f'
- Floating point decimal format.
- 'F'
- Floating point decimal format.
- 'g'
- Floating point format. Uses lowercase exponential format if exponent is less than -4 or not less than precision, decimal format otherwise.
- 'G'
- Floating point format. Uses uppercase exponential format if exponent is less than -4 or not less than precision, decimal format otherwise.
- 'c'
- Single character (accepts integer or single character string).
- 'r'
- String (converts any Python object using repr().
- 's'
- String (converts any Python object using str()
- '%'
- No argument is converted, results in a '%' character in the result.
Date Formatting
Date Formatting
- %a
- Abbreviated weekday (Sun)
- %A
- Weekday (Sunday)
- %b
- Abbreviated month name (Jan)
- %B
- Month name (January)
- %c
- Date and time
- %d
- Day (leading zeros) (01 to 31)
- %H
- 24 hour (leading zeros) (00 to 23)
- %I
- 12 hour (leading zeros) (01 to 12)
- %j
- Day of year (001 to 366)
- %m
- Month (01 to 12)
- %M
- Minute (00 to 59)
- %p
- AM or PM
- %S
- Second (00 to 61?)
- %U
- Week number1 (00 to 53)
- %w
- Weekday2 (0 to 6)
- %W
- Week number3 (00 to 53)
- %x
- Date
- %X
- Time
- %y
- Year without century (00 to 99)
- %Y
- Year (2008)
- %Z
- Time zone (GMT)
- %%
- A literal '%' character (%)
Released:
Emojis for Python
Project description
Emojis for Python
About
This library allows you to emojify content such as:This is a message with emojis :smile: :snake:
Emoji database is based on gemojilibrary.
See the Emoji cheat sheet formore examples.
Installation
Install emojis with pip.
pip3 install -U emojis
Missing or wrong emoji?
This library is based on gemojidatabase, the official GitHub emoji library for Ruby.
If an emoji is missing or labeled wrongly, report togemoji project. If gemoji fix it,this library will be updated as soon as there’s a new gemoji release.
All issues complaining about missing emojis will be closed.
All PRs to include custom emojis will be closed.
Project details
Release historyRelease notifications | RSS feed
0.6.0
0.5.1
0.5.0
0.4.0
0.3.1
0.3.0
Python Syntax Cheat Sheet Pdf
0.2.0
0.1.0
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size emojis-0.6.0-py3-none-any.whl (27.7 kB) | File type Wheel | Python version py3 | Upload date | Hashes |
Filename, size emojis-0.6.0.tar.gz (27.3 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for emojis-0.6.0-py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | 7da34c8a78ae262fd68cef9e2c78a3c1feb59784489eeea0f54ba1d4b7111c7c |
MD5 | ba1c4b0d433cc5173a0ee50d1940f3c3 |
BLAKE2-256 | 2e9461025e53488acd95b49862ec854e05b036f92fe9d0e512ca551a5a8b03d6 |
Hashes for emojis-0.6.0.tar.gz
Python Cheat Sheet Code With Mosh
Algorithm | Hash digest |
---|---|
SHA256 | bf605d1f1a27a81cd37fe82eb65781c904467f569295a541c33710b97e4225ec |
MD5 | f5dc6c7174fee189e558eb9a71cf1b46 |
BLAKE2-256 | 276387db5712e9c030e48d99a55f06623d48feb38a1adfacc027b0d4ce928a2d |
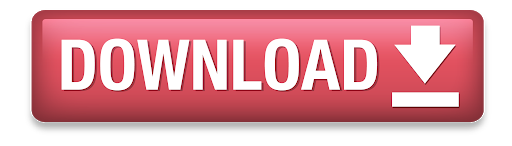