If you are interested in performance, you need to know more about CUDA.
From the official website:
CUDA® is a parallel computing platform and programming model developed by NVIDIA for general computing on graphical processing units (GPUs). With CUDA, developers are able to dramatically speed up computing applications by harnessing the power of GPUs.
In GPU-accelerated applications, the sequential part of the workload runs on the CPU – which is optimized for single-threaded performance – while the computing-intensive portion of the application runs on thousands of GPU cores in parallel. When using CUDA, developers program in popular languages such as C, C++, Fortran, Python, and MATLAB and express parallelism through extensions in the form of a few basic keywords.
Nsight Visual Studio Edition CUDA Debugger Source Code View. View correlated Source, PTX, and SASS. Set breakpoints in Source and/or SASS. Step over, in, out, continue in source or PTX/SASS disassembly. Conditional breakpoints operate on source vars and PTX/SASS regs. Hover over variables and registers to. J-a-lewis changed the title Work With nVidia So VS Code Can Be Used To Setup CUDA For Machine Learning Instead of Visual Studio Community Work With nVidia So VS Code Can Be Used To Setup CUDA For Machine Learning Instead of Having To Use Visual Studio Community May 26, 2020.
In this post, I will guide you through your first steps with CUDA. Also, I will show you how to move some basic processing from the CPU to the GPU.
Let’s start getting some bytes.
Developer community 2. Search Search Microsoft.com. Visual Studio Code is a code editor redefined and optimized for building and debugging modern web and cloud applications. Visual Studio Code is free and available on your favorite platform. Browse other questions tagged c cuda visual-studio-code vscode-settings or ask your own question. The Overflow Blog Podcast 332: Non-fungible Talking. The Loop: Our Community & Public Platform Roadmap for Q2 2021. Featured on Meta Stack Overflow for.
Environment setup
I am using Visual Studio 2017 (version 15.6.0). To code with CUDA, you will need to download and install the CUDA Toolkit. The Toolkit includes Visual Studio project templates and the NSight IDE (which it can use from Visual Studio).
Also, you will need to install the VC++ 2017 toolset (CUDA is still not compatible with the latest version of Visual Studio).
Starting a new project using CUDA
The easiest way to start a project that uses CUDA is utilizing the CUDA template.
To be able to compile this, you will need to change the Project Properties to use the Visual Studio 2015 toolset.
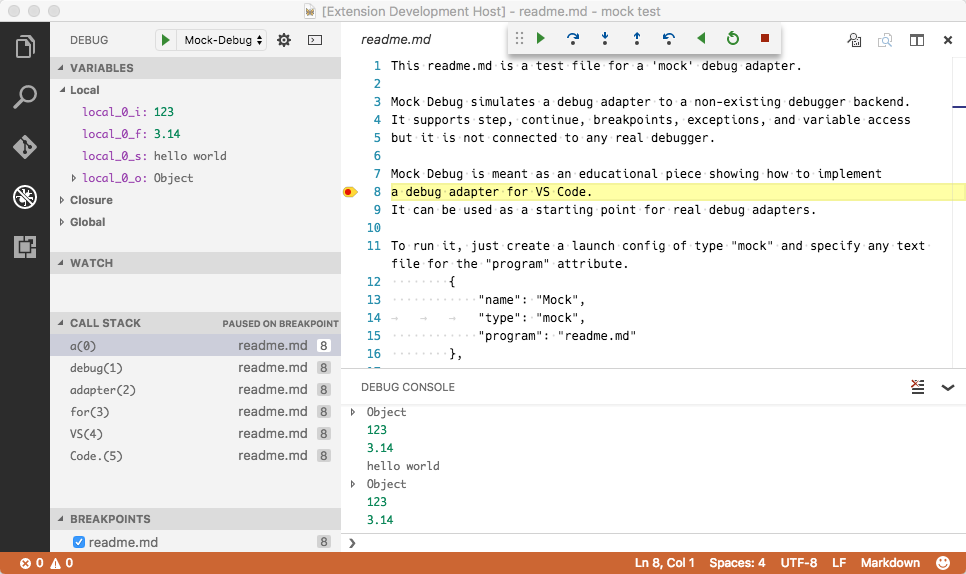
I recommend you to clean the template’s boilerplate changing the content of the file kernel.cu to this:
Cuda Vs2017 Msb3721
Basic CPU processing
Let’s add two arrays (a and b) putting the results in a third array (c).
This code is pretty simple. But it is tough to parallelize.
Whenever we want to use parallelism, we need to create functions that could be executed independently. The function add_arrays_cpu has a for-loop that runs the add process in a sequential fashion. But, there is no reason to work in this way. Let’s change it:
The function add_array_element_cpu can be executed independently, and that is great. We could start a thread for each position of the array, and that would work fine (I am not saying that would be the right thing to do with CPUs, just as an example).
Bringing CUDA to the game
The next logical step is to start using the GPU to run our code. We will not move all the functions to the GPU, but only the functions we think we could be parallelized.
We marked the add_arrays_gpu function as __global__. Using CUDA terminology, this function is a kernel, and that will be executed from the GPU. It runs on the GPU, called from the CPU (there is another qualifier location qualifier, __device__ that should be used with functions that run on GPU, called from the GPU).
__global__ functions are invoked using the special <<<…>>> syntax. The parameters we used indicates that we are running the kernel function in 1 block of count (5) threads. We will talk more about it in the future.
Note that we don’t need to inform an index anymore – we retrieve the index position from threadIdx.x. threadIdx is a special variable, provided by CUDA runtime, that informs the position of the current thread in the thread block (we are using five threads, one thread block to run our code. Because of that, we were able to use this information as the index in the array).
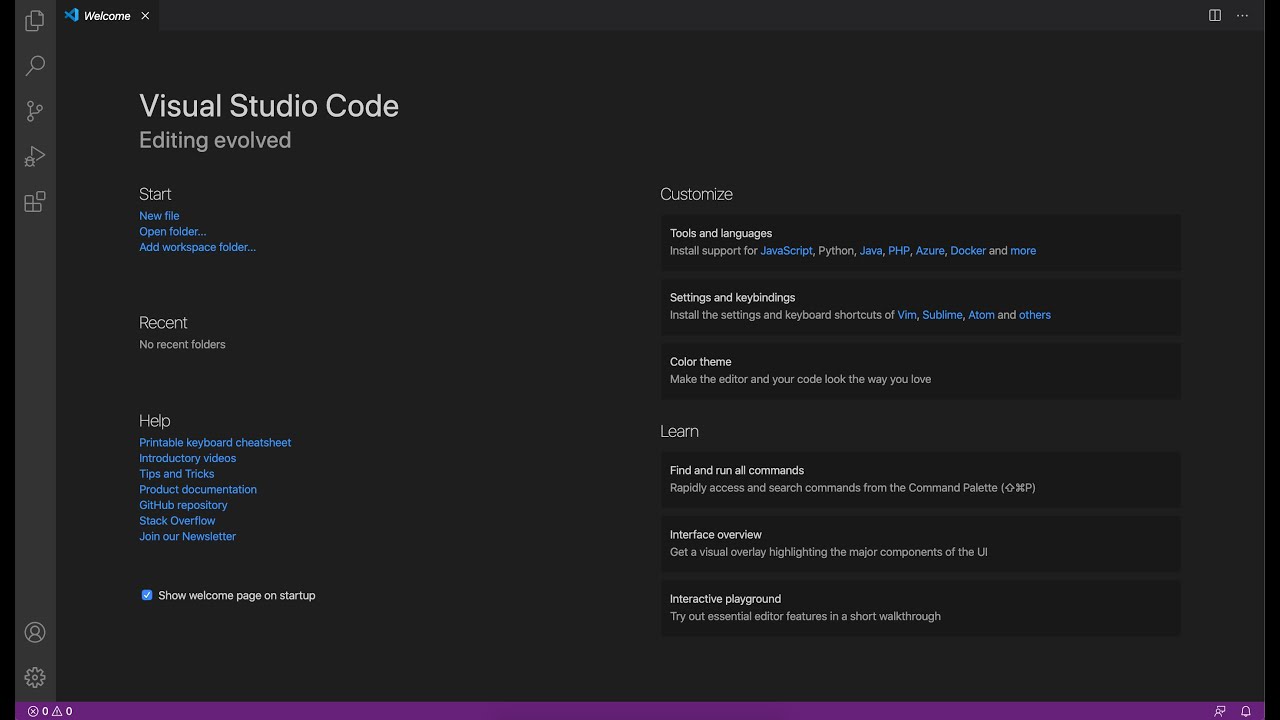
I would like to make a special consideration about a vital pattern that you will see a lot of times when doing CUDA programming. To run, GPU code is not allowed to access CPU memory (and vice-versa). So we will need:
- copy the data we need to process from the CPU to the GPU memory
- do the processing
- copy results from the GPU to the CPU memory.

Final words
Cuda Without Visual Studio
I know, I know! Adding two arrays with only five elements each is not an exciting example. But, in this post, we did the setup to use CUDA on our machines. Then, I helped you to move your could from sequential running on CPU to parallel on GPU. In the future, let’s return to it and get some real benefits.
Cover image: Jean Gerber
In this blog, I want to show users how to set up vs-code for CUDA C/C++ code in Windows 10.
Introduction

I’ve been working on cuda programming in Visual Studio, which can be set up easily. However, since I play with vs-code, I would like to use vs-code for cuda as well. So In this blog, I want to show users how to set up vs-code for cuda in Windows.
There are some major steps you need to take, in order to run/debug cuda code using vs-code.
- (Optional, if done already) Enable Linux Bash shell in Windows 10 and install vs-code in Windows 10.
- Download the extension in vs-code: vscode-cudacpp. It is mainly for syntax and snippets.
- Download the sample code from my GitHub repository.
- Press Ctrl+Shift+B in vs-code, choose build to compile the code. Choose run to run the executable.
- Currently it is not able to enable cuda-debugger for cuda in vs-code in Windows. If you were to do everything in bash, then there might be a possibility to configure cuda-debugger.
- But it is OK to use Windows C/C++ debugger, to only debug CPU code.
c_cpp_properties.json
tasks.json
launch.json
Visual Studio Code Cuda-gdb
Reference:
Cuda With Visual Studio Code
- [1] .
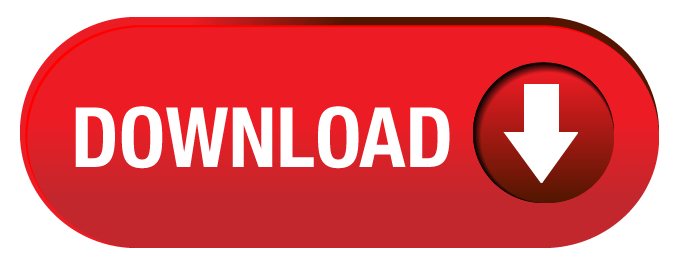