As a developer, you probably know that parsing C++ source code is very complicated. This is also the reason why there might be fewer good tools for coding assistance than we have in other “lighter” languages. Fortunately, parsing C++ source code has become much more straightforward over the past decade, mainly because of the Clang/LLVM infrastructure. Clang not only introduced a new excellent C++ compiler but also many extra tools that allow us to analyse C++ code efficiently.
- How To Compile In Vs Code
- C Programming In Vs Code
- Clang Tidy Visual Studio
- Visual Studio Code Clang-tidy
- Visual Studio Code Clang Format
- Visual Studio Code Clang Windows
- Visual Studio Code Clang Compiler
Search results for 'clang', Visual Studio Code on marketplace.visualstudio.com.
- Launch.json 'version': '0.2.0', 'configurations': 'name': '(gdb) Launch', 'type': 'cppdbg'.
- Using Clang in Visual Studio Code In this tutorial, you configure Visual Studio Code on macOS to use the Clang/LLVM compiler and debugger. After configuring VS Code, you will compile and debug a simple C program in VS Code. This tutorial does not teach you about Clang or the C language.
Note: You can use the C toolset from Visual Studio Build Tools along with Visual Studio Code to compile, build, and verify any C codebase as long as you also have a valid Visual Studio license (either Community, Pro, or Enterprise) that you are actively using to develop that C codebase. Today, under Windows, we will use VS Code to compile and link C code with Clang. Windows 10 is running; Install VS Code. In April 2018, I use 1.21.1. Launch VS Code and then install the C/C for Visual Studio Code extension. Use the extension icon on the left or press CTRL+SHIFT+X; Install LLVM. In April 2018 you should install LLVM.
Today we’ll meet one tool that can make our life much easier! It’s called clang-tidy.
We’ll cover this valuable utility as it’s also an important part for the Visual Assist internal code analysis system.
Let’s Meet clang-tidy
Here’s a concise and a brief description of this handy tool:
clang-tidy is a clang-based C++ “linter” tool. Its purpose is to provide an extensible framework for diagnosing and fixing typical programming errors, like style violations, interface misuse, or bugs that can be deduced via static analysis. clang-tidy is modular and provides a convenient interface for writing new checks.
As you can read above, this tool gives us a way to check our source code and fix common issues.
Let’s now see how you can install and work with this tool on Windows.
Installation on Windows
clang-tidy is attached with the main Clang package for Windows. You can download it from this site:
(Or also see other Releases: Download LLVM releases)
When Clang is installed, you can open your command line (for example, for this article I’m using PowerShell) and type:
I get the following output:
In general, clang-tidy operates on a single input file. You pass a filename, plus standard compilations flags and then extra flags about selected checks that will be performed.
Let’s run a simple test to see how it works.
Running a Simple Test
See this artificial code:
Can you now play a game and list all of the “spurious” smells in the code? What would you improve here?
Let’s now compare your results with suggestions from clang-tidy.
I ran the tool with the following options:
On my machine, I get the those results:
As you can see above, I run it against all checks in the “modernise” and “readability” categories. You can find all available checks here: clang-tidy – Clang-Tidy Checks — Extra Clang Tools 12 documentation
You can also list all available checks for your version with the following command:
In summary the tool found the following issues:
- suggestion to use trailing return type for main()
- suggestion about using .empty() rather than comparing string with an empty string
- make_unique
- nullptr rather than NULL
- modernising range based for loop
- braces around single-line if and loop statements
Experimenting with Fixes
But there’s more!
clang-tidy can not only find issues but also fix them! A lot of checks have “auto-fixes”! By providing the -fix
command, you can ask the tool to modify the code. For example:
As you can see, this time I used “readability-container” only as I didn’t want to modify braces around simple if statements.
I got the following output:
clang-tidy nicely lists all the fixes that it managed to apply.
The final source code looks as follows:
In the above code sample the tool managed to fix several issues, and we can decide if we want to apply all or maybe just select a few of them. For example, I’m not sure about using a trailing return type for all functions. Additionally, the tool couldn’t improve and apply make_unique
in the place where we declare and initialise ptr
. So hopefully, with each new revision, we’ll get even better results.
But also it’s important to remember that you have to be careful with the fixes.
See lines 1 and 12.
Clang-tidy added extra and duplicated header file “memory” (this was probably needed for make_unique()). This is not critical, but shouldn’t happen. But what’s worse is that at line 12 the code is now wrong.
The line if (hello + world ')
was changed into if (hello + world.empty())
. This changes the meaning of the statement!
When applying fixes, be sure to review code and check if this is what you expected.
I hope you now get a general idea of how to use the tool and what its output is.
Running this tool from a command line might not be the best choice. Fortunately, there are many options on how we can plug it inside our build system or IDE.
For example, you can learn how to make use of it with CMake on a KDAB blog or… Clang-Tidy, part 1: Modernise your source code using C++11/C++14 – KDAB
Let’s now have a brief look at the options in Visual Studio.
Clang Tools in Visual Studio
clang-tidy support is available starting with Visual Studio 2019 version 16.4.
clang-tidy is the default analysis tool when using the LLVM/clang-cl toolset, but you can also enable it with MSVC compiler:
And you can configure it with a separate property panel:
And when you run a code analysis you can get results to your Error/Warning list:
Unfortunately, the current version of Visual Studio doesn’t offer a way to apply fixes, so you need to modify the code on your own.
How To Compile In Vs Code
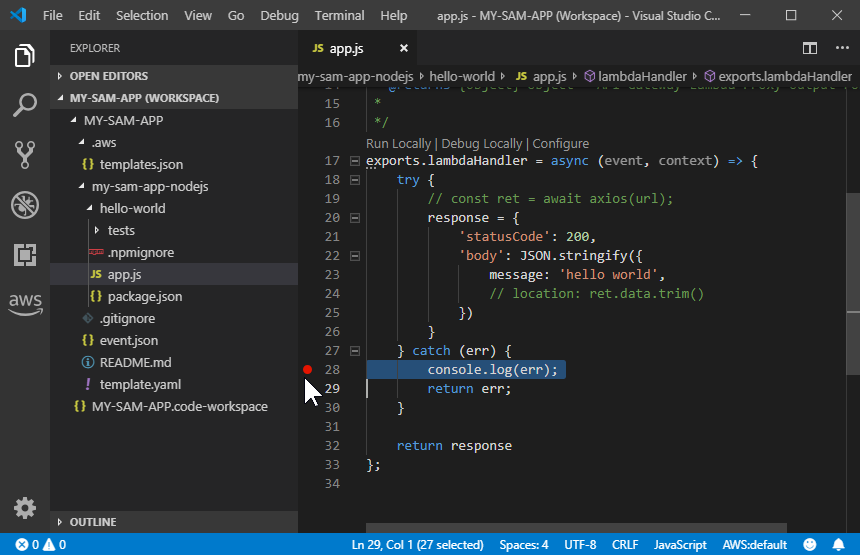
C Programming In Vs Code
Luckily, with the help of Visual Assist, you can change it. See below.
How Visual Assist Makes Things Much Safer And Easier
Visual Assist offers a service called “Code Inspection”, which is based on a standalone LLVM/Clang embedded into the VA system. You can enable it (even in Visual studio 2008!), and for our simple example you might get the following results (in a separate VA Code Inspection Window):
And what’s best is that for many of them you can apply a fix!
See the following context menu:
This is great! Thanks to the embedded LLVM/Clang subsystem, Visual Assist can perform the analysis and help you with many tasks related to code modernisation and fundamental code analysis!
But what’s best is that Visual Assist cleans up the output from clang-tidy and makes sure the fixes are correct and safe. Here’s the code after I applied all suggestions:
Nice!
As you can see there’s no extra include statement. And what’s most important is in line 12. VA added extra brackets, so the whole expression is now correct and safe!
Summary
In this article, we covered clang-tidy – a handy tool for code analysis that can (experimentally) fix your code automatically! The tool is quite verbose and might be hard to configure to work with large projects. In addition, please make sure you review code when applying fixes.
By default, you can download it and launch from a command line, but it’s much better to use it from Visual Studio (limited).
To get the best experience and safety have a look at the embedded clang-tidy inside Visual Assist – in the form of “VA Code Inspections”. This extra feature makes sure the results of code analysis are easy to read and meaningful, and the fixes are correct.
Today we only scratched the surface of this exciting topic. In two upcoming blog posts you’ll see some more use cases where Visual Assist can help you with code refactoring and modernisation (also leveraging embedded clang-tidy). Stay tuned.
For now you can read more in:
- Introduction to Code Inspection Visual Assist Documentation
This page gives you the shortest path to checking out Clang and demos a fewoptions. This should get you up and running with the minimum of muss and fuss.If you like what you see, please consider gettinginvolved with the Clang community. If you run into problems, please filebugs in LLVM Bugzilla.
Clang Tidy Visual Studio
Release Clang Versions
Visual Studio Code Clang-tidy
Clang is released as part of regular LLVM releases. You can download the release versions from https://llvm.org/releases/.
Clang is also provided in all major BSD or GNU/Linux distributions as part of their respective packaging systems. From Xcode 4.2, Clang is the default compiler for Mac OS X.
Visual Studio Code Clang Format
Building Clang and Working with the Code
On Unix-like Systems
If you would like to check out and build Clang, the current procedure is asfollows:
- Get the required tools.
- See Getting Started with the LLVM System - Requirements.
- Note also that Python is needed for running the test suite. Get it at: https://www.python.org/downloads/
- Standard build process uses CMake. Get it at: https://cmake.org/download/
- Check out the LLVM project:
- Change directory to where you want the llvm directory placed.
- git clone https://github.com/llvm/llvm-project.git
- Build LLVM and Clang:
- cd llvm-project
- mkdir build (in-tree build is not supported)
- cd build
- cmake -DLLVM_ENABLE_PROJECTS=clang -G 'Unix Makefiles' ../llvm
- make
- This builds both LLVM and Clang for debug mode.
- Note: For subsequent Clang development, you can just run make clang.
- CMake allows you to generate project files for several IDEs: Xcode, Eclipse CDT4, CodeBlocks, Qt-Creator (use the CodeBlocks generator), KDevelop3. For more details see Building LLVM with CMake page.
- If you intend to use Clang's C++ support, you may need to tell it how to find your C++ standard library headers. In general, Clang will detect the best version of libstdc++ headers available and use them - it will look both for system installations of libstdc++ as well as installations adjacent to Clang itself. If your configuration fits neither of these scenarios, you can use the -DGCC_INSTALL_PREFIX cmake option to tell Clang where the gcc containing the desired libstdc++ is installed.
- Try it out (assuming you add llvm/build/bin to your path):
- clang --help
- clang file.c -fsyntax-only (check for correctness)
- clang file.c -S -emit-llvm -o - (print out unoptimized llvm code)
- clang file.c -S -emit-llvm -o - -O3
- clang file.c -S -O3 -o - (output native machine code)
- Run the testsuite:
- make check-clang
Using Visual Studio
Visual Studio Code Clang Windows
The following details setting up for and building Clang on Windows usingVisual Studio:
- Get the required tools:
- Git. Source code control program. Get it from: https://git-scm.com/download
- CMake. This is used for generating Visual Studio solution and project files. Get it from: https://cmake.org/download/
- Visual Studio 2017 or later
- Python. It is used to run the clang test suite. Get it from: https://www.python.org/download/
- GnuWin32 tools The Clang and LLVM test suite use various GNU core utilities, such as grep, sed, and find. The gnuwin32 packages are the oldest and most well-tested way to get these tools. However, the MSys utilities provided by git for Windows have been known to work. Cygwin has worked in the past, but is not well tested. If you don't already have the core utilies from some other source, get gnuwin32 from http://getgnuwin32.sourceforge.net/.
- Check out LLVM and Clang:
- git clone https://github.com/llvm/llvm-project.git
Note: Some Clang tests are sensitive to the line endings. Ensure that checking out the files does not convert LF line endings to CR+LF. If you're using git on Windows, make sure your core.autocrlf setting is false.
- Run CMake to generate the Visual Studio solution and project files:
- cd llvm-project
- mkdir build (for building without polluting the source dir)
- cd build
- If you are using Visual Studio 2017: cmake -DLLVM_ENABLE_PROJECTS=clang -G 'Visual Studio 15 2017' -A x64 -Thost=x64 ..llvm
-Thost=x64 is required, since the 32-bit linker will run out of memory. - To generate x86 binaries instead of x64, pass -A Win32.
- See the LLVM CMake guide for more information on other configuration options for CMake.
- The above, if successful, will have created an LLVM.sln file in the build directory.
- Build Clang:
- Open LLVM.sln in Visual Studio.
- Build the 'clang' project for just the compiler driver and front end, or the 'ALL_BUILD' project to build everything, including tools.
- Try it out (assuming you added llvm/debug/bin to your path). (See the running examples from above.)
- See Hacking on clang - Testing using Visual Studio on Windows for information on running regression tests on Windows.
Using Ninja alongside Visual Studio
We recommend that developers who want the fastest incremental builds use theNinja build system. You can use thegenerated Visual Studio project files to edit Clang source code and generate asecond build directory next to it for running the tests with these steps:
- Check out clang and LLVM as described above
- Open a developer command prompt with the appropriate environment.
- If you open the start menu and search for 'Command Prompt', you should see shortcuts created by Visual Studio to do this. To use native x64 tools, choose the one titled 'x64 Native Tools Command Prompt for VS 2017'.
- Alternatively, launch a regular cmd prompt and run the appropriate vcvarsall.bat incantation. To get the 2017 x64 tools, this would be:
'C:Program Files (x86)Microsoft Visual Studio2017CommunityVCAuxiliaryBuildvcvarsall.bat' x64
- mkdir build_ninja (or build, or use your own organization)
- cd build_ninja
- set CC=cl (necessary to force CMake to choose MSVC over mingw GCC if you have it installed)
- set CXX=cl
- cmake -GNinja ..llvm
- ninja clang This will build just clang.
- ninja check-clang This will run the clang tests.
Visual Studio Code Clang Compiler
Clang Compiler Driver (Drop-in Substitute for GCC)
The clang tool is the compiler driver and front-end, which isdesigned to be a drop-in replacement for the gcc command. Here aresome examples of how to use the high-level driver:
The 'clang' driver is designed to work as closely to GCC as possible to maximize portability. The only major difference between the two is that Clang defaults to gnu99 mode while GCC defaults to gnu89 mode. If you see weird link-time errors relating to inline functions, try passing -std=gnu89 to clang.
Examples of using Clang
